
What are the errors in below code? What will be output and how can you fix it?
<?php
$arr = array();
$arr['var1'] = array(1, 2);
$arr['var2'] = 3;
$arr['var3'] = array(4, 5);
$test = array();
$test = array_merge($test, $arr['var1']);
var_dump($test);
$test = array_merge($test, $arr['var2']);
var_dump($test);
$test = array_merge($test, $arr['var3']);
var_dump($test);
?>
Last updated 4 years, 10 months ago | 1510 views 75 5
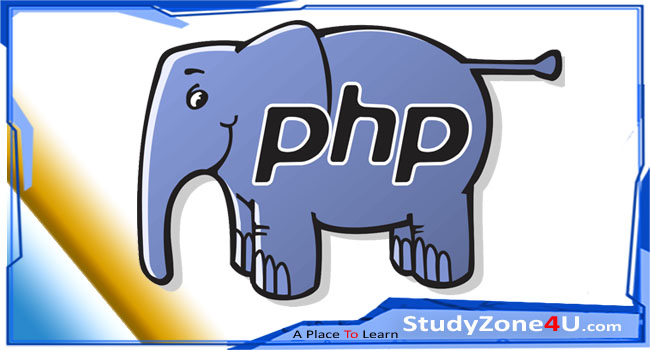
PHP | Array error fix | array_merge()
The output will be as follows:
array(2) { [0]=> int(1) [1]=> int(2) } NULL NULL
It will also generate two warning messages as follows:
Warning: array_merge(): Argument #2 is not an array Warning: array_merge(): Argument #1 is not an array
As a result, when there's a call to $test = array_merge($test, $arr['var2']) evaluates to array_merge($test, 3) and, since 3 is not an array and will returns null. This will set $test to null. As $test is now null, then next call to the $test = array_merge($test, $arr['var3']) again return null.
(This also explains why the first warning complains about argument #2 and the second warning complains about argument #1.)
To fix the code we need to typecast the second argument to an array as follows:
<?php
$test = array_merge($test, (array)$arr['var1']);
var_dump($test);
$test = array_merge($test, (array)$arr['var2']);
var_dump($test);
$test = array_merge($test, (array)$arr['var3']);
var_dump($test);
?>
which will generate the following output:
array(2) { [0]=> int(1) [1]=> int(2) } array(3) { [0]=> int(1) [1]=> int(2) [2]=> int(3) } array(5) { [0]=> int(1) [1]=> int(2) [2]=> int(3) [3]=> int(4) [4]=> int(5) }