
Why JQuery Ajax is sending GET instead of POST
Last updated 5 years, 10 months ago | 8980 views 75 5
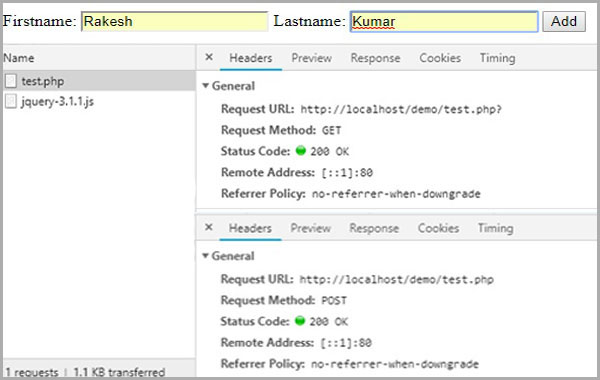
JQuery | Ajax is sending GET instead of POST
It's a very common problem which is faced by most of the new programer. In Ajax code, new learners use (type: "POST") yet the sending request went through GET instead of POST.
Let's see how the problem can be short out.
<?php
$conn = mysqli_connect("localhost","root","","test");
if (!$conn) {
die("Connection failed: " . mysqli_connect_error());
}
if(isset($_POST['add'])){
$firstname=$_POST['firstname'];
$lastname=$_POST['lastname'];
mysqli_query($conn,"insert into `user` (firstname, lastname) values ('$firstname', '$lastname')");
}
?>
<!DOCTYPE html>
<html lang = "en">
<head>
<title>JQuery Ajax is sending GET instead of POST</title>
</head>
<body>
<br>
<form>
<label>Firstname:</label>
<input type = "text" id = "firstname">
<label>Lastname:</label>
<input type = "text" id = "lastname">
<button type = "submit" id="addnew">Add</button>
</form>
<script src = "js/jquery-3.1.1.js"></script>
<script type = "text/javascript">
$(document).ready(function(){
$(document).on('click', '#addnew', function(){
var f_name=$('#firstname').val();
var l_name=$('#lastname').val();
$.ajax({
type: "POST",
url: "test.php",
data: {
firstname: f_name,
lastname: l_name,
add: 1,
},
success: function(){
showUser();
}
});
});
});
</script>
</body>
</html>
Above code produce General header status with GET request instead we use (type: "POST") in our ajax code.
Request URL: http://localhost/demo/test.php? Request Method: GET Status Code: 200 OK Remote Address: [::1]:80 Referrer Policy: no-referrer-when-downgrade
This is not happening because of the Ajax code. Our Ajax code is right but the problem in our HTML code. In the above code, we use a form with a button that is used to send the form data. If we see the attribute of our button then we find it uses two attributes (i.e.: type="submit" id="addnew").
<button type = "submit" id="addnew">Add</button>
Button type = "submit" has the properties to send any form data instantly to the HTTP Header. By default, it sends data by GET request if you do not define any method type in the form attribute.
So for resolving the problem we just have to change button type = "button" instead of type = "submit".
<button type = "button" id="addnew">Add</button>
If we change button type = "button" then we see the code produce General header status with POST request.
Request URL: http://localhost/demo/test.php Request Method: POST Status Code: 200 OK Remote Address: [::1]:80 Referrer Policy: no-referrer-when-downgrade
In this way, we can fix the problem of "JQuery Ajax is sending GET instead of POST".